The lightning/navigation service is used to navigate in Lightning Experience, Lightning Communities and in Salesforce Application. Navigation Service in Lightning Web Components are used to navigate to Record Pages, Web Pages, Objects, List Views, Custom Tabs, Related Lists, Files etc.
Navigation Service uses a PageReference rather than a URL. A PageReference is a JavaScript object that provides the details of Page Type, attributes and state of the Page.
The PageReference envelopes a component from future changes to URL formats. Page type(String) and attributes(Object) are required parameters, state(Object) is optional parameter. To use the navigation service in Lightning Web Components, first import it in the JavaScript file,
import { NavigationMixin } from 'lightning/navigation';
Next apply NavigationMixin function to component’s base class
export default class SampleNavigationService extends NavigationMixin(LightningElement) {
NavigateMixin adds two API’s to component’s class,
- NavigateMixin.Navigate : To navigate to another page in the application
- NavigateMixin.GenerateURL : To get a promise that resolves to the resulting URL. The URL can be used in the href attribute of an anchor. It can utilize the URL to open a new window using the Browser api – Window.open(url)
Example of Navigation Service in Lightning Web Components
Please find below the code snippet that provides an insight on how Navigation Service is used to navigate to New Case record creation on click of ‘New Case’ button:
sampleNavigationService.html
<template>
<lightning-card title="Navigation Service">
<div class="slds-p-left_medium">
<lightning-button label="New Case" onclick={navigateToNewCasePage}></lightning-button>
</div>
</lightning-card>
</template>
sampleNavigationService.js
import { LightningElement } from 'lwc';
import { NavigationMixin } from 'lightning/navigation';
export default class SampleNavigationService extends NavigationMixin(LightningElement) {
navigateToNewCasePage() {
this[NavigationMixin.Navigate]({
type: 'standard__objectPage',
attributes: {
objectApiName: 'Case',
actionName: 'new'
},
});
}
}

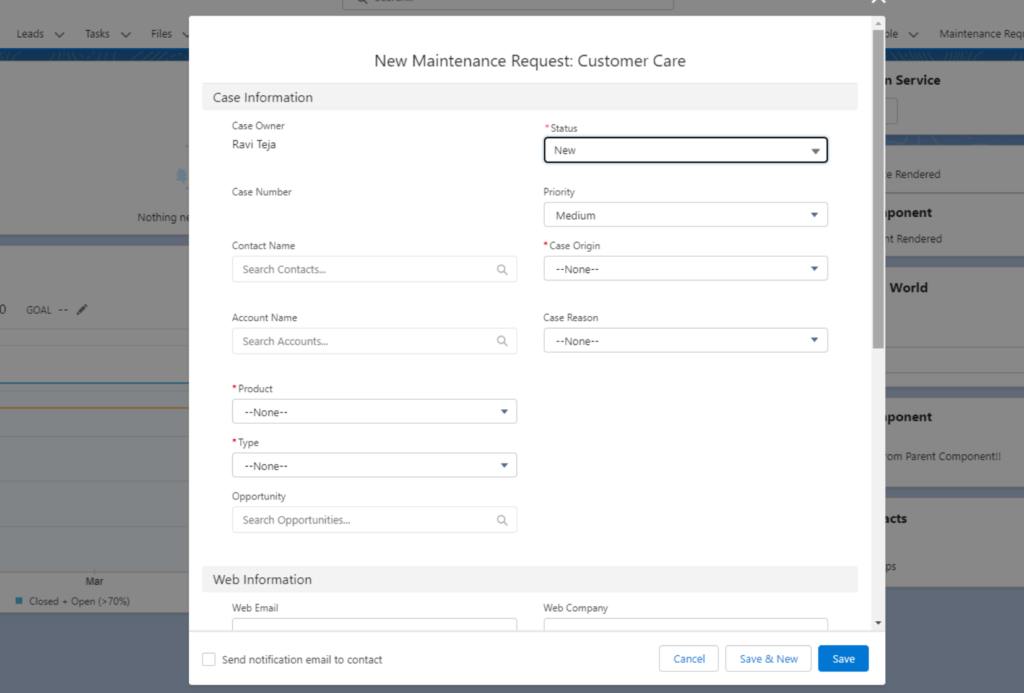
How to Navigate to Case Home Page
Please find below the code snippet that provides an insight on basic Navigation Service that is used to navigate to Case Home Page on click of ‘Case Home’ URL:
basicNavigationLWC.html
<template>
<lightning-card title="Case Home Page Navigation">
<div class="slds-p-left_medium">
<a href={refUrl} onclick={handleNavigationClick}>Case Home</a>
</div>
</lightning-card>
</template>
basicNavigationLWC.js
import { LightningElement, wire } from 'lwc';
import { NavigationMixin } from 'lightning/navigation';
export default class BasicNavigationLWC extends NavigationMixin(LightningElement) {
refUrl;
connectedCallback() {
this.caseHomePageRef = {
type: 'standard__objectPage',
attributes: {
objectApiName: 'Case',
actionName: 'home'
}
};
this[NavigationMixin.GenerateUrl](this.caseHomePageRef)
.then(url => this.refUrl = url);
}
handleNavigationClick(evt) {
evt.preventDefault();
evt.stopPropagation();
this[NavigationMixin.Navigate](this.caseHomePageRef);
}
}
Navigation to record page
navigateToViewCasePage() {
this[NavigationMixin.Navigate]({
type: 'standard__recordPage',
attributes: {
recordId: this.recId,
objectApiName: 'Case',
actionName: 'view'
},
});
}
Navigation to custom application
navigateToMyCustomApplication() {
this[NavigationMixin.Navigate]({
type: 'standard__app',
attributes: {
appTarget: 'c__MyCustomApplication',
}
});
}
Navigation to external URL
navigateToApexHoursPage() {
this[NavigationMixin.Navigate]({
type: 'standard__webPage',
attributes: {
url: 'https://www.apexhours.com/'
}
});
}
Navigation to custom tab
navigateToCustomTab() {
this[NavigationMixin.Navigate]({
type: 'standard__navItemPage',
attributes: {
apiName: 'CustomTabName'
},
});
}
Navigation to List View
navigateToCaseListView() {
this[NavigationMixin.Navigate]({
type: 'standard__objectPage',
attributes: {
objectApiName: 'Case',
actionName: 'list'
},
state: {
filterName: 'Recent'
},
});
}
Navigation to Related List
navigateToContactRelatedList() {
this[NavigationMixin.Navigate]({
type: 'standard__recordRelationshipPage',
attributes: {
recordId: this.recordId,
objectApiName: 'Account',
relationshipApiName: 'Contacts',
actionName: 'view'
},
});
}
Navigation to Files
navToFilesPage() {
this[NavigationMixin.Navigate]({
type: 'standard__objectPage',
attributes: {
objectApiName: 'ContentDocument',
actionName: 'home'
},
});
}
Navigation to Tab
navigateToCustomTab() {
this[NavigationMixin.Navigate]({
type: 'standard__navItemPage',
attributes: {
apiName: 'CustomTabName'
},
});
}
Navigation to Visualforce Page
navigateToVisualForcePage() {
this[NavigationMixin.GenerateUrl]({
type: 'standard__webPage',
attributes: {
url: '/apex/CaseVFExample?id=' + this.recordId
}
}).then(generatedUrl => {
window.open(generatedUrl);
});
}
Navigation to Custom Aura Component
openCustomLightningComponent(){
this[NavigationMixin.Navigate]({
type: 'standard__component',
attributes: {
componentName: 'c__AuraComponentName'
}
});
}