User Experience is an essential element of any application and Salesforce is no exception to this. In this episode, we will explore the programmatic capability of the platform to build custom user experiences using Visualforce page in salesforce. Join us as you embark on this wonderful journey to become a champion Salesforce developer.
What is Visualforce page in salesforce?
Visualforce is Markup language that allows you to describe the User Interface which can live on your Salesforce Platform. Visualforce pages consist of two elements: Visualforce markup, and a controller.

MVC Pattern
Let learn about MVP pattern in Visualforce page.

Visualforce Markup
- Visualforce Tags
- Over 150+ Standard Components
- Ability to build Custom Components
- Works with all standard web Technologies
- CSS
- JavaScript
- HTML
Decode Standard Component
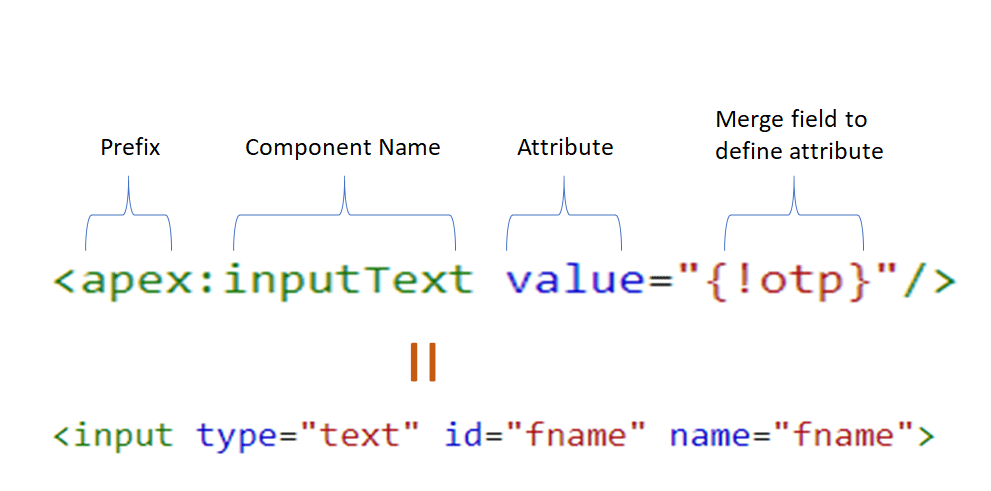
1. Standard Controller in Visualforce page
- Controller is class which define action needs to be taken based User interaction with view.
- Each Salesforce Object (Standard/Custom) always comes with Controller which is know as Standard Controller.
- Basic operations of sObject are already implemented in Standard Controller like,
- Save
- Delete
- Cancel
- Edit
2. Custom Controller in Visualforce page
- A custom controller is an Apex class that implements all of the logic for a page without leveraging a standard controller
- Use custom controllers when you want your Visualforce page to run entirely in system mode, which does not enforce the permissions and field-level security of the current user
3. Controller Extension
- A controller extension is an Apex class that extends the functionality of a standard or custom controller.
- When to use controller extensions?
- You want to leverage the built-in functionality of a standard controller but override one or more actions.
- You want to add new actions.
- You want to build a Visualforce page that respects user permissions. Although a controller extension class executes in system mode, if a controller extension extends a standard controller, the logic from the standard controller does not execute in system mode.
Visualforce page Order of Execution
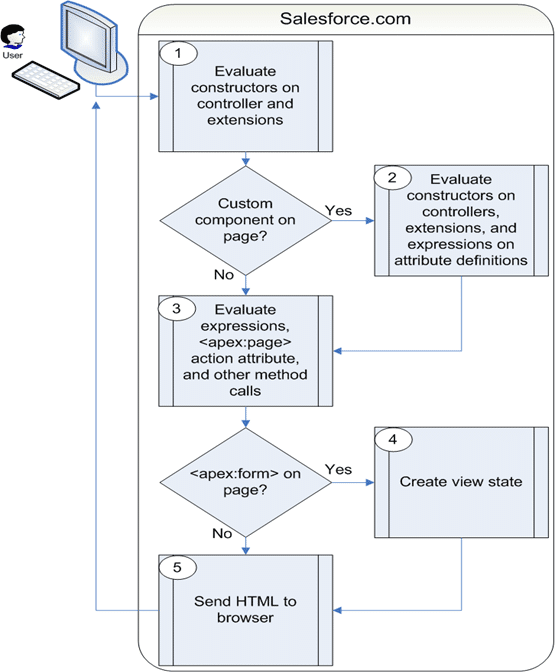
Visualforce page in salesforce Considerations
- Unless a class has a method defined as webService, custom extension and controller classes and methods are generally defined as public. If a class includes a web service method, it must be defined as global.
- Use sets, maps, or lists when returning data from the database. This makes your code more efficient because the code makes fewer trips to the database.
- The Apex governor limits for Visualforce controller extensions and custom controllers are the same as the limits for anonymous block or WSDL methods. For more information about governor limits, see Execution Governors and Limits in the Appendix.
- If you are building a custom controller or controller extension, be careful that you do not inadvertently expose sensitive data that would normally be hidden from users. Consider using the with sharing keywords on class definitions to enforce permissions. Also be careful using Web services, which are secured as top-level entry points by the profile, but execute in the system context once they are initiated.
- Apex methods and variables are not instantiated in a guaranteed order. For more information, see Getting and Setting Data with a Custom Extension or Controller.
- You can’t use data manipulation language (DML) operations in a “getxxx” method in a controller. For example, if your controller had a getName method, you could not use insert or update in the method to create an object.
- You can’t use data manipulation language (DML) operations in a constructor method in a controller.
- You can’t use the @future annotation in a “getxxx” or “setxxx” method in a controller, or in the constructor for a controller.
- Primitive Apex data types such as String or Integer are passed by value to the component’s controller.
- Non-primitive Apex data types such as lists and sObjects are passed by reference to component’s controller. This means that if component’s controller changes the name of an account, the changes are available in page’s controller.
Recording
Further Learning
Assignment
Complete below assignment to win $1000 Salesforce Voucher. Click here for rule.
•Create Visualforce Page with called Calculator. Which will perform arithmetic operations and show result •Page should display 2 input box which are accepting 2 different user input in form of integer. •Define 4 custom actions Add, Subtract, Multiply and divide. •Add 4 buttons on Visualforce page which will perform respective action. For example button add will perform addition of 2 numbers. •Add Output field on page which will display output of action performed. Hint : Use reRender attribute to display output. |
Don’t forget to register for our next session on Introduction to AURA. Check this post for all other session detail.
Please note that we have limit of 500 attendees that can join the online sessions. However, recording will be posted on our YouTube channel. Make sure to subscribe our YouTube channel to get notification for video upload.
So, learn at your pace and free will and ace your journey to Salesforce!
Completed Assignment for Day 10
Completed Assignment on Visualforce page
Glad you like our Visualforce page in salesforce session
Assignment done
Assignment 10 Completed
Solution:
VISUALFORCE PAGE ;-
—————————————————-
APEX CLASS –
public class CalculatorApex {
public Boolean showOutput {get;set;}
public Integer number1 {get;set;}
public Integer number2 {get;set;}
public Integer output {get;set;}
public void CalculatorApex(){
number1 = 0;
number2 = 0;
output = 0;
showOutput = false;
}
public void Add(){
output = number1 + number2;
showOutput = true;
}
public void Subtract(){
output = number1 – number2;
showOutput = true;
}
public void Multiply(){
output = number1 * number2;
showOutput = true;
}
public void Divide(){
if ( number2 == 0 ) {
ApexPages.addMessage(new ApexPages.Message(ApexPages.Severity.WARNING, ‘Value cannot be zero’));
}
else {
output = number1 / number2;
showOutput = true;
}
}
}
Code for Assignment Day 10
——————————–
——————————————————————————————-
public class CalculatorController {
Integer firstValue;
Integer secondValue;
Integer result;
Double resultDouble;
String resultMessage=’Default message’;
public Boolean rend { get; set; }
public Integer getFirstValue() {
return firstValue;
}
public void setFirstValue(Integer f1){
firstValue = f1;
}
public Integer getSecondValue(){
return secondValue;
}
public void setSecondValue(Integer f2){
secondValue=f2;
}
public String getResultMessage(){
return resultMessage;
}
public PageReference doAdd(){
rend = true;
if(firstValue == 0) {
resultMessage = ‘Please enter First Value or should not be 0:’;
}else if(secondValue == 0 ) {
resultMessage = ‘Please enter Second Value or should not be 0:’;
} else {
result = firstValue + secondValue;
resultMessage = ‘Addition of two numbers is:’ + result;
}
return null;
}
public PageReference dosubStraction(){
rend = true;
if(firstValue == 0) {
resultMessage = ‘Please enter First Value or should not be 0:’;
} else if(secondValue == 0) {
resultMessage = ‘Please enter Second Value or should not be 0:’;
} else {
result = firstValue – secondValue;
resultMessage = ‘Subtraction of two numbers is:’ + result;
system.debug(‘resultMessage:’ + resultMessage);
}
return null;
}
public PageReference doMultiplication(){
rend = true;
if(firstValue == 0) {
resultMessage = ‘Please enter First Value or should not be 0:’;
}else if(secondValue == 0) {
resultMessage = ‘Please enter Second Value or should not be 0:’;
} else {
result = firstValue * secondValue;
resultMessage = ‘Multiplication of two numbers is:’ + result;
system.debug(‘resultMessage:’ + resultMessage);
}
return null;
}
public PageReference doDivision(){
rend = true;
if(firstValue == 0) {
resultMessage = ‘Please enter First Value or should not be 0:’;
}else if(secondValue == 0) {
resultMessage = ‘Please enter Second Value or should not be 0:’;
} else {
resultDouble = (double) firstValue / (double)secondValue;
resultMessage = ‘Division of two numbers is:’ + resultDouble;
system.debug(‘resultMessage:’ + resultMessage);
}
return null;
}
}