Exception are unexpected event occurred during your transaction execution. Let see Different types of Exceptions in Salesforce and how to solve them with Salesforce best practices. In this post we will cover all common error in Salesforce.
What is Exception in Salesforce?
Exceptions note errors and other events that disrupt the normal flow of code execution. throw statements are used to generate exceptions, while try, catch, and finally statements are used to gracefully recover from exceptions.
Different Type of Exceptions in Salesforce
Here are some common exceptions and error in Apex. Follow Salesforce Apex Best practices to avoid below exceptions and errors. Let see how to resolve the different Type of Exceptions in Salesforce.
1. DmlException
Any problem with a DML statement, such as an insert statement missing a required field on a record, Mixed DML and etc.
1.1. Required Field Missing
It throw the System.DmlException: Insert failed. First exception on row 0; first error: REQUIRED_FIELD_MISSING
exception.
try {
Contact cont = new Contact();
cont.FirstName ='Apex';
insert cont;
} catch(DmlException e) {
System.debug('Exception is : ' + e.getMessage());
}
Solution: Include all record field before performing DML event.
1.2. Mixed DML Operation Error
A mixed DML Operation Error comes when you try to perform DML operations on setup and non-setup objects in a single transaction. It will give you System.DmlException: Insert failed. First exception on row 0; first error: MIXED_DML_OPERATION
error.
Solution: Check our Mixed DML Operation Error in Salesforce post for solution.
1.3. Invalid Data
System.DmlException: Insert failed. First exception on row 0 with id XXX; first error: INVALID_FIELD_FOR_INSERT_UPDATE, cannot specify Id in an insert call: [Id]
. This issue come while inserting a same record in Trigger.
Solution: Don’t include Id while inserting the record.
2. System.FinalException
2.1. Record is Read only
“System.FinalException: Record is read-only
” error come when you are trying to edit a Trigger.new
record in after insert/update trigger. The records are read only in that context as they have been written, but not committed, to the database.
Solution: Update record in before event or query record before updating it from database.
3. ListException
Any problem with a list, such as attempting to access an index that is out of bounds will throw System.ListException: List index out of bounds
Exception
try {
List <Account> listAccounts = [ SELECT Id FROM Account WHERE Name = 'ApexHours' ];
System.debug( 'Name is ' + listAccounts.get( 0 ).Name );
} catch(ListException e) {
System.debug('Exception is : ' + e.getMessage());
}
Solution: Use size function to avoid the List out of bound issue.
4. NullPointerException
Any problem with dereferencing null, such as in the following code:
try {
String s;
Boolean b = s.contains('Apex');
} catch(NullPointerException e) {
System.debug('Exception is : ' + e.getMessage());
}
It will throw the “System.NullPointerException: Attempt to de-reference a null object
” error.
Solution: Allocate memory or assign value before using the object. Learn more.
5. QueryException
Any problem with SOQL queries, such as assigning a query that returns no records or more than one record to a singleton sObject variable.
5.1. No rows for assignment
Here is example of “ System.QueryException: List has no rows for assignment to SObject“
try {
Account a = [SELECT Name FROM Account WHERE Name = 'XYZ' LIMIT 1];
} catch(QueryException e) {
System.debug('Exception is : ' + e.getMessage());
}
Solution: Use list to avoid this issue.
5.2. System.QueryException: Non-selective query against large object type
If you query records on object that returns more than 200,000 records and without query filters, it’s possible that you’ll receive the error, “System.QueryException: Non-selective query against large object type.
Solution: Learn SOQL best practice to fix this issue.
6. SObjectException
Any problem with sObject records, such as attempting to change a field in an update statement that can only be changed during insert.
6.1. SObject row was retrieved via SOQL without querying
Below is example of System.SObjectException: SObject row was retrieved via SOQL without querying the requested field: Account.Name
.
try {
Account a = [SELECT Id FROM Merchandise__c LIMIT 1];
System.debug('-->'+a.name);
} catch(Exception e) {
System.debug('Exception is : ' + e.getMessage());
}
Solution: Include field in SOQL query
7. LimitException
In Salesforce we can encounter the Salesforce Governor Limits. A governor limit has been exceeded. This exception can’t be caught. This is a hard limit, means you can’t increase it by contacting Salesforce support.
Here are some common Limit exceptions.
7.1. Too Many SOQL Queries: 101 error
This means we can only have up to 100 SOQL in a single context. This is a hard limit, which means you can’t increase it by contacting Salesforce support. Here is example.
For(Account acc: Trigger.new){
for(Contact con:[select id from contact where accountId = :acc.Id]){
}
}
Solution: Check how to Resolve System.limitException: Too many SOQL Queries 101.
7.2. Too many DML statements: 151
You can not perform more then 150 DML statements in one execution.
for(Account acc : accList)
{
Account accNew = new Account();
accNew.Id = acc.id;
Update accNew;
}
Solution: So what you need to do is, you need to create a list of all the records and insert that list using the DML statement, in this way you can insert multiple records only in one DML statement.
7.3. Apex CPU Time Limit Exceeded
Salesforce CPU time limits usage to 10 seconds for synchronous transactions and 60 seconds for asynchronous processing. The “Apex CPU time limit exceeded” error means your transaction is taking too long and can’t be completed.
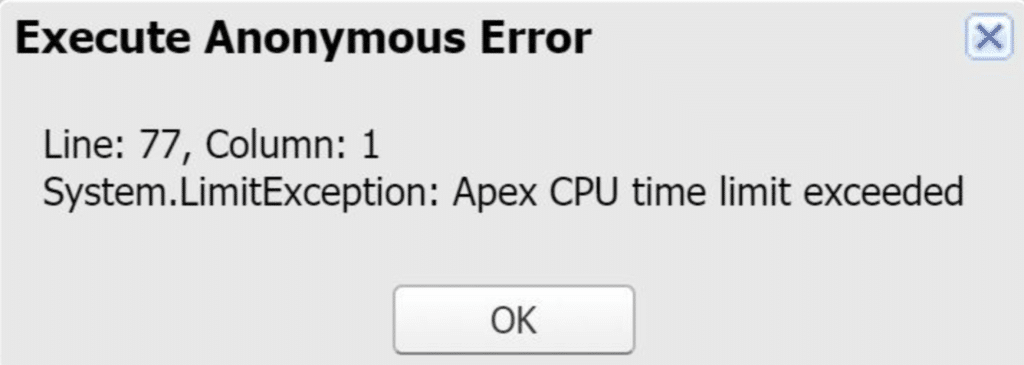
Solution: Check solution on Apex CPU Time Limit Exceeded post.
7.4. Too many Query rows
You will get LimitException: Too many query rows: 50001
error when your query will return more then 50,000 record.
Solution: Use Limit and filter in your SOQL query.
8. StringException
Any problem with Strings, such as a String that is exceeding your heap size.
Here is example of “System.StringException: Invalid Id
“.
Solution: ” isn’t a valid Id. Use null instead.
9. JSONException
Any problem with JSON serialization and deserialization operations. For more information, see the methods of System.JSON, System.JSONParser, and System.JSONGenerator.
9.1. Apex Type unsupported in JSON: Object
JSON.deserialize(String, Type)
method cannot deserialize from JSON into generic Object
, or into objects with generic Object
.
Solution: Use Wrapper class to deserialize the Json. Learn more here.
10. UnexpectedException
A non-recoverable internal error within Salesforce has occurred. This exception causes execution to halt. If necessary, contact Salesforce Customer Support for more information.
Solution: System.UnexpectedException is usually followed by a error message. Code should handle the conditions that can cause this exception and to prevent it in advance, but cannot be handled once it is generated. Error message is usually self-descriptive, but if it has a Salesforce error code, please contact salesforce.com support.
11. FlowException
Any problem with starting flow interviews from Apex. For example, if an active version of the flow can’t be found or it can’t be started from Apex.
Solution: Learn about how to handle exception in flow.
Exception Logging In Salesforce
Check our post to learn how to Exception Handling logger framework using Platform Events.
Summary
There are more Exceptions and error in Salesforce. We will update this list soon with more exceptions and common error. Let us know which one is missing in this list.
Images used in email not render in my email client (gmail).
Might be your gmail account exception 🙂