A Batch Apex in Salesforce allows you to define a single job that can be broken up into manageable chunks that will be processed separately. Batch Apex is a global class that implements the Database.Batchable interface. In this post, we will learn about what is Batch Apex and when to use Batch Apex example.
What is Batch Apex in Salesforce?
The Batch class is used to process millions of records within normal processing limits. With Batch Apex, we can process records asynchronously to stay within platform limits. If you have a lot of records to process, for example, data cleansing or archiving, Batch Apex is probably your best solution. In Batch Apex each transaction starts with a new set of governor limits, making it easier to ensure that your code stays within the governor execution limits.
When to use Batch Apex
Here is the real-time scenario to use the Apex Batch job.
- If you want to send an email 90 days before the Contract expiration, we want to notify the User that the Contract is going to expire.
- Create a renewal opportunity base on contract end date.
- Batch Apex is also used to recalculate Apex Sharing for large data volume.
Batch Job Execution
Let understand how batch job execution happened and in which order all method execute.
1) Start method is identifies the scope (list of data to be processed) and automatically called at the beginning of the apex job. This method will collect record or objects on which the operation should be performed.
global Database.QueryLocator start(Database.BatchableContext BC){}
2) Execute Method processes a subset of the scoped records and performs operation which we want to perform on the records fetched from start method.
global void execute(Database.BatchableContext BC, list<sobject<) {}
3) Finish method executes after all batches are processed. This method use for any post job or wrap-up work like send confirmation email notifications.
global void finish(Database.BatchableContext BC) {}
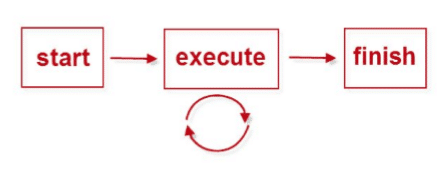
Batch Apex Example In Salesforce
Let take one example for batch job in Salesforce. If you need to make a field update to every Account in your organization. If you have 10,001 Account records in your org, this is impossible without some way of breaking it up.
- So in the start() method, you define the query you’re going to use in this batch context ‘select Id from Account’.
- Then the execute() method runs, but only receives a relatively short list of records (default 200). Within the execute(), everything runs in its own transactional context, which means almost all of the governor limits only apply to that block. Thus each time execute() is run, you are allowed 150 queries and 50,000 DML rows and so on. When that execute() is complete, a new one is instantiated with the next group of 200 Accounts, with a brand new set of governor limits.
- Finally the finish() method wraps up any loose ends as necessary, like sending a status email.
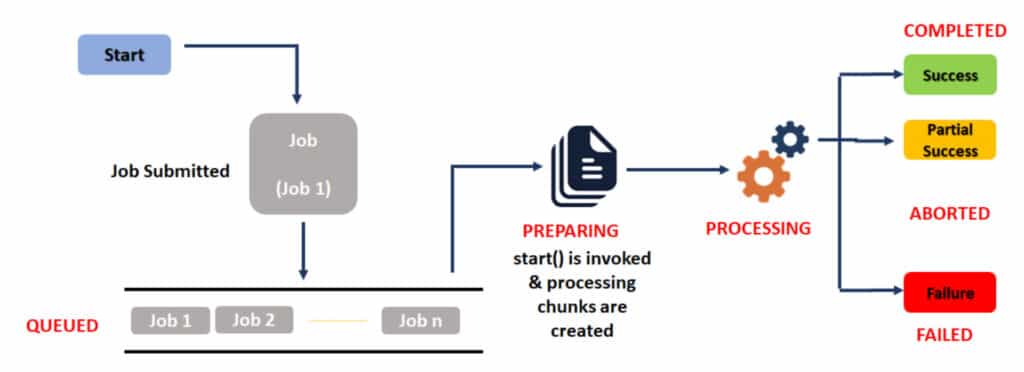
Sample Batch Job
here is sample batch job.
global class AccountUpdateBatchJob implements Database.Batchable<sObject>
{
global Database.QueryLocator start(Database.BatchableContext BC)
{
String query = 'SELECT Id,Name FROM Account';
return Database.getQueryLocator(query);
}
global void execute(Database.BatchableContext BC, List<Account> scope)
{
for(Account a : scope)
{
a.Name = a.Name + 'Updated by Batch job';
}
update scope;
}
global void finish(Database.BatchableContext BC) {
}
}
Using Callouts in Batch Apex
To do the callout in batch Apex, use Database.AllowsCallouts
in the class definition. Like below example.
public class SearchAndReplace implements Database.Batchable<sObject>,
Database.AllowsCallouts{
}
What is stateful in the batch apex
Batch Apex is stateless by default. If a batch process needs information that is shared across transactions, then you need to implement a Stateful interface. If you specify Database.Stateful in the class definition, you can maintain state across these transactions.
For example, if your batch job is executing one logic and you need to send an email at end of the batch job with all successful records and failed records. For that, you can use Database.Stateful in the class definition.
public class MyBatchJob implements
Database.Batchable<sObject>, Database.Stateful{
public integer summary;
public MyBatchJob(String q){
Summary = 0;
}
public Database.QueryLocator start(Database.BatchableContext BC){
return Database.getQueryLocator(query);
}
public void execute(
Database.BatchableContext BC,
List<sObject> scope){
for(sObject s : scope){
Summary ++;
}
}
public void finish(Database.BatchableContext BC){
}
}
How to execute a Batch job?
You can execute a batch job with DataBase.executeBatch(obj,size)
method or we can schedule a batch job with scheduler class. Let see how to execute a batch job
AccountUpdateBatchJob obj = new AccountUpdateBatchJob();
DataBase.executeBatch(obj);
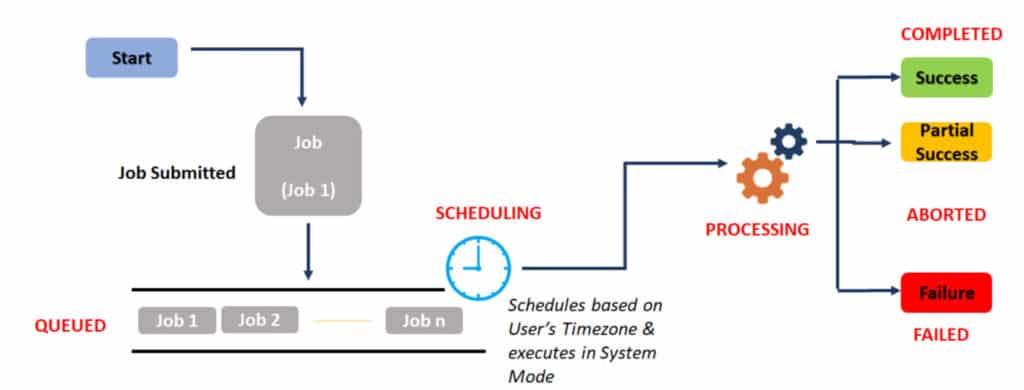
Scheduler Class For Batch Apex
Schedulable Class is a global class that implements the Schedulable interface. That includes one execute method. Here is example of a scheduler class
global class AccountUpdateBatchJobscheduled implements Schedulable {
global void execute(SchedulableContext sc) {
AccountUpdateBatchJob b = new AccountUpdateBatchJob();
database.executebatch(b);
}
}
How to Schedule scheduler class
There are two option we have schedule the scheduler classes.
1) By System Scheduler.
2) By Developer console
System Scheduler
Step 1) Click on Setup->Apex class. Then search Schedule Apex button.

Step 2) Select the scheduler class and set Time like below screen shot.

By Developer console
Execute below code from developer console.
AccountUpdateBatchJobscheduled m = new AccountUpdateBatchJobscheduled();
String sch = '20 30 8 10 2 ?';
String jobID = system.schedule('Merge Job', sch, m);
Test Class for Batch Job
How to write test class for Batch Job.
@isTest
public class AccountUpdateBatchJobTest {
static testMethod void testMethod1() {
List<Account> lstAccount= new List<Account>();
for(Integer i=0 ;i <200;i++) {
Account acc = new Account();
acc.Name ='Name'+i;
lstLead.add(acc);
}
insert lstAccount;
Test.startTest();
AccountUpdateBatchJob obj = new AccountUpdateBatchJob();
DataBase.executeBatch(obj);
Test.stopTest();
}
}
Limits in Batch Apex
- Up to five queued or active batch jobs are allowed for Apex.
- Cursor limits for different Force.com features are tracked separately. For example, you can have 50 Apex query cursors, 50 batch cursors, and 50 Visualforce cursors open at the same time.
- A maximum of 50 million records can be returned in the Database.QueryLocator object. If more than 50 million records are returned, the batch job is immediately terminated and marked as Failed.
- If the start method returns a QueryLocator, the optional scope parameter of Database.executeBatch can have a maximum value of 2,000. If set to a higher value, Salesforce chunks the records returned by the QueryLocator into smaller batches of up to 2,000 records. If the start method returns an iterable, the scope parameter value has no upper limit; however, if you use a very high number, you may run into other limits.
- If no size is specified with the optional scope parameter of Database.executeBatch, Salesforce chunks the records returned by the start method into batches of 200, and then passes each batch to the execute method.Apex governor limits are reset for each execution of execute.
- The start, execute, and finish methods can implement up to 10 callouts each
- The maximum number of batch executions is 250,000 per 24 hours.
- Only one batch Apex job’s start method can run at a time in an organization. Batch jobs that haven’t started yet remain in the queue until they’re started. Note that this limit doesn’t cause any batch job to fail and execute methods of batch Apex jobs still run in parallel if more than one job is running.
Summary
In this post we learn about what is Batch Apex in Salesforce and when we should use Batch Apex. I hope this helped you to learn how batch class is used to process millions of records with in normal processing limits.
Very Good Salesforce Blogs
Glad this batch apex post helped you
Nice post. It helps my team in development.
Glad this Batch apex post helped you
Subcutaneous Injections
Looking for a 1BHK flat in Talegaon Dabhade? Discover exceptional living spaces with Clover Group. Our premium residential offerings in Talegaon Dabhade combine modern comfort and serene surroundings.
https://clovercasablanca.in/
Looking for your dream home? Discover affordable 2 BHK flats under 40 lakhs in Pune with Clover Group.
https://clovercasablanca.in/
Looking for your dream home? Discover affordable 2 BHK flats under 40 lakhs in Pune with Clover Group. Experience modern living, prime locations, and excellent amenities.
https://clovercasablanca.in/
Great explanation !!!
Hi,
Nice Post !
I have a request, can you please check point 6 of ( Limit in Batch Apex) as you have mentioned that 10 Callouts are possible but i have read in developer.salesforce website that limit is of 100.
link:- https://developer.salesforce.com/docs/atlas.en-us.apexcode.meta/apexcode/apex_batch_interface.htm
Thanks & Regards
Naveen Tiwary