Apex is a powerful programming language used in Salesforce for developing custom applications and automating business processes. This tutorial aims to provide beginners with a solid understanding of the core fundamentals of Apex coding, including key concepts and syntax. Let’s start the Understanding the Core Fundamentals of Apex for Salesforce: A Starter’s Tutorial.
Key Takeaways
- Variables and Data Types are fundamental building blocks in Apex coding.
- Control Structures help in controlling the flow of execution in Apex code.
- Exception Handling is crucial for managing errors and unexpected situations in Apex programs.
- Methods and Classes are essential components for organizing code in Apex development.
- Triggers and Governor Limits play a significant role in governing the behavior and limits of Apex code execution.
Key Concepts of Apex Coding
Here are Fundamentals of Apex for Salesforce: A Starter’s Tutorial.
Variables and Data Types
In Apex, variables are the basic units of storage that you can define, store, and manipulate within your programs. Variables are named containers for storing data values. Apex is a statically typed language, which means that the type of a variable must be declared before it can be used. This helps in preventing errors, such as type mismatches, at compile time rather than at runtime.
Data types in Apex can be primitive, such as Integer, Double, Boolean, and String, or they can be more complex, like sObjects for Salesforce records and collections such as Lists, Sets, and Maps. Understanding these data types and their appropriate usage is crucial for effective Apex programming.
Here is a brief overview of some common Apex data types:
- Primitive Data Types: These include basic types like Integer, Long, Double, Decimal, Boolean, String, and Date.
- sObject: Represents Salesforce records and is used to query and manipulate data stored in Salesforce objects.
- Collections: Include List (ordered collection), Set (unordered collection of unique elements), and Map (collection of key-value pairs).
Choosing the right data type for a variable is essential for both performance and clarity of your code. For instance, when dealing with a large number of unique values, a Set would be more appropriate than a List to avoid duplicates and to allow for faster searches.
Control Structures
Control structures are fundamental in Apex programming as they dictate the flow of execution within your code. Conditional statements like if, else if, and else, along with switch statements, allow your program to execute different code blocks based on certain conditions. Looping structures such as for, while, and do-while loops enable repetitive execution of code blocks until a specified condition is met.
Here’s a brief overview of common control structures in Apex:
- if statement: Executes a block of code if a specified condition is true.
- else clause: Follows an if statement and executes a block of code if the if condition is false.
- else if clause: Provides a secondary condition if the first if condition is false.
- switch statement: Selects one of many code blocks to be executed.
- for loop: Iterates over a sequence of values or a range of numbers.
- while loop: Repeats a block of code while a specified condition is true.
- do-while loop: Executes a block of code once, and then repeats the loop as long as a specified condition is true.
Understanding and effectively utilizing control structures is crucial for creating efficient and readable Apex code. They are the building blocks that help manage the logic and flow of your applications, ensuring that the right code is executed at the right time.
Exception Handling
Exception handling in Apex is a critical aspect of writing robust and error-resistant code. It ensures that your application can gracefully handle unexpected situations without crashing or exhibiting unpredictable behavior. Apex provides a structured way to catch and handle exceptions using try, catch, and finally blocks.
When an exception occurs, the Apex runtime searches for a catch block that matches the exception type. If a match is found, the code within that catch block is executed, allowing for error processing and recovery. If no catch block is found, the exception propagates up the call stack, which can lead to unhandled exceptions and potential system failures.
Here is a simple structure of how exception handling works in Apex:
- Try Block: Contains the code that may throw an exception.
- Catch Block: Catches and handles the exception. Multiple catch blocks can be used to handle different types of exceptions.
- Finally Block: Executes code after the try and catch blocks, regardless of whether an exception was thrown or caught. It is typically used for resource cleanup.
Proper exception handling is essential for maintaining the integrity and reliability of your Apex applications. It allows developers to control the flow of the program and provides a mechanism for error reporting and logging, which is invaluable for debugging and maintaining code.
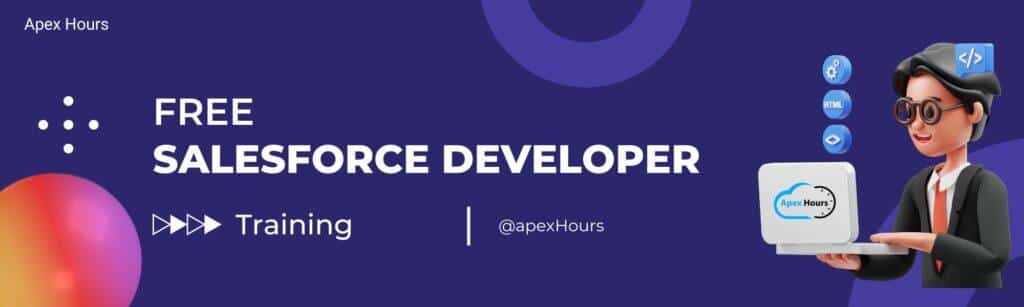
Understanding Apex Syntax
Methods and Classes
In Apex, methods are blocks of code that perform specific tasks and can be called upon when needed, enhancing code reusability and organization. Classes, on the other hand, are blueprints for creating objects that encapsulate data and methods, providing structure to your Apex code.
When defining a class, you typically include both attributes (variables) and behaviors (methods). For instance, a Student class might have attributes like name and studentId, and behaviors such as enrollInCourse() or displaySchedule().
Here’s a simple breakdown of a class structure in Apex:
- Attributes: Define the properties of the class.
- Methods: Define the actions the class can perform.
- Constructor: A special method used to create and initialize objects from the class.
Understanding the relationship between methods and classes is crucial for effective Apex programming. Methods should be designed to be as independent and reusable as possible, while classes should be coherent and focused on representing a single concept or entity.
Triggers
In Apex, triggers are powerful pieces of code that execute before or after data manipulation language (DML) events occur. These events include operations such as insert, update, delete, and undelete on Salesforce records. Apex Triggers allow developers to perform custom actions and enforce business logic, ensuring data integrity and automating processes within the Salesforce platform.
Triggers can be categorized based on when they execute in relation to the DML event:
- Before Triggers: Execute before the records are saved to the database. They are typically used for validation and setting default values.
- After Triggers: Execute after the records have been saved to the database. They are used for accessing field values that are set by the system and for affecting changes in other records.
It’s crucial to understand the context of trigger execution to avoid common pitfalls. Here’s a simple table outlining the context variables available in triggers:
Variable | Description |
isExecuting | Indicates if the current context is a trigger. |
isInsert | Indicates if the trigger was fired due to an insert operation. |
isUpdate | Indicates if the trigger was fired due to an update operation. |
isDelete | Indicates if the trigger was fired due to a delete operation. |
isBefore | Indicates if the trigger is a before trigger. |
isAfter | Indicates if the trigger is an after trigger. |
new | Provides the new version of the sObject records. |
old | Provides the old version of the sObject records. |
Developers must also be mindful of the governor limits in Salesforce, which restrict the amount of resources a trigger can consume during its execution. This ensures that the shared resources on the Salesforce platform are used efficiently and that one process does not monopolize the system.
Governor Limits
Understanding Governor Limits is crucial when developing in Apex, as Salesforce enforces these runtime limits to ensure shared resources are used efficiently across the multi-tenant environment. These limits prevent individual Apex code from monopolizing shared resources, which could negatively impact the overall performance of the Salesforce platform.
Governor Limits cover various aspects of the code execution and API usage, such as the number of SOQL queries, DML statements, and future method calls that can be made in a single transaction. Here’s a brief overview of some common Governor Limits:
- Total number of SOQL queries issued
- Total number of DML statements executed
- Maximum CPU time on the Salesforce servers
- Total heap size
It’s important for developers to be aware of these limits and design their code to handle bulk operations efficiently. Exceeding these limits will result in a runtime exception, which can disrupt the user experience. Therefore, developers should employ best practices such as bulkifying code, using efficient queries, and implementing proper error handling to avoid hitting these limits.
Best Practices in Apex Development
Code Optimization
In the realm of Apex development, code optimization is crucial for creating efficient, maintainable, and scalable applications. By adhering to optimization best practices, developers can significantly reduce code execution time and resource consumption, which is particularly important given the Governor Limits in Salesforce.
One fundamental approach to optimizing Apex code is to minimize the number of database operations. This can be achieved by bulkifying code to handle multiple records simultaneously, thus reducing the number of queries and DML operations. Additionally, developers should leverage collections such as Lists, Maps, and Sets to efficiently process data in memory.
Another key aspect is the efficient use of loops and conditional statements. Avoiding unnecessary iterations and ensuring that queries are outside of loops can have a substantial impact on performance. Here’s a simple guideline to follow:
- Bulkify your code to handle multiple records at once.
- Use collections to manage data more efficiently.
- Place SOQL queries outside of loops.
- Apply conditional logic judiciously to avoid redundant processing.
By implementing these strategies, developers can create optimized Apex code that adheres to Salesforce’s best practices and delivers optimal performance.
Testing Strategies
In Apex development, testing is not an afterthought but a core part of the development process. Salesforce enforces a strict requirement that all custom code must have a significant percentage of test coverage before it can be deployed to a production environment. This ensures that your code is robust and can handle various scenarios without failing.
Effective testing strategies involve more than just achieving the required code coverage; they include writing tests that validate the logic of your code and simulate user behaviors and scenarios. Here are some key points to consider when developing your testing strategies:
- Write unit tests that cover positive, negative, and edge cases.
- Use test setup methods to create test data and contexts.
- Employ mocking frameworks to simulate external systems and dependencies.
- Ensure that your tests are independent of each other and can run in any order.
- Regularly run your tests during development to catch issues early.
By adhering to these strategies, you can build a suite of tests that not only meet Salesforce’s requirements but also contribute to the overall quality and maintainability of your Apex code.
Security Considerations
When developing in Apex, security is not an afterthought but a fundamental aspect that must be woven into the fabric of your code. Always enforce object- and field-level permissions to ensure that users can only access the data they are authorized to view or manipulate. This is crucial for maintaining the integrity and confidentiality of data within Salesforce.
To systematically address security concerns, consider the following checklist:
- Validate all inputs to prevent injection attacks.
- Use sharing rules and profiles to manage data access.
- Employ encryption for sensitive data both at rest and in transit.
- Regularly review and update security settings in response to new threats.
Remember, security is a continuous process that evolves with your application and the broader threat landscape. By adhering to these practices and staying informed about the latest security developments, you can help protect your Salesforce environment from potential vulnerabilities.
Frequently Asked Questions
The key concepts include variables and data types, control structures, and exception handling.
The syntax includes methods and classes, triggers, and governor limits.
Code optimization involves improving the efficiency and performance of your Apex code.
Governor Limits are restrictions set by Salesforce to ensure efficient resource utilization in Apex code execution.
Testing ensures the functionality and reliability of your Apex code before deployment.
Security considerations involve protecting sensitive data, preventing vulnerabilities, and complying with security standards.
Conclusion
In conclusion, mastering the core fundamentals of Apex for Salesforce is essential for beginners looking to dive into the world of Salesforce development. By understanding key concepts and syntax, beginners can build a strong foundation for creating powerful applications on the Salesforce platform. This starter’s tutorial aims to demystify Apex coding and provide a clear pathway for beginners to enhance their skills and knowledge in Salesforce development. With dedication and practice, beginners can unlock the full potential of Apex and embark on a rewarding journey in Salesforce development. I hope this will help you to Understand the Core Fundamentals of Apex for Salesforce.
Author Details
Gobinath A
Co-Founder & Chief Marketing Officer