In this article, I will tell you how to check object permission using Apex, but before that, let me explain why we need to check object permission using code.
Suppose you are writing an Apex class that involves database operations or a query. Apex code generally runs in a system context, which means the permissions of current logged-in users aren’t considered during code execution. If you don’t check permissions, a user without permission will be able to make database changes or query. Hence, as a best practice, you must respect the object permissions of logged-in users.
How to check object permission from setup
Before jumping into the code, let’s see how to check object permission from setup.
Follow the steps below to check the object permission from setup.
- Go to Profiles.
- Select the profile for which you want to see the permission.
- Scroll down to Standard Object Permissions if you want to check permission of standard object else scroll to Custom Object Permissions.
- Here you can see the Read, Write, Edit, Delete permissions for the profile which you have selected in step 2.
Check Object Permission Using Apex
So we have learned how to check object permissions from setup; now let’s learn how to do the same using Apex. Execute the code snippet below from the Anonymous Window.
//To check the object-level permission for the Account before creating the account
if(Schema.SobjectType.Account.iscreateable()){
System.debug('User has permission to create Account Records');
}
//To check the object-level permission for the Account before updating the account
if(Schema.SobjectType.Account.isupdateable()){
System.debug('User has permission to update Account Records');
}
//To check the object-level permission for the Account before deleting the account
if(Schema.SobjectType.Account.isdeletable()){
System.debug('User has permission to delete Account Records');
}
//To check the object-level permission for the Account before querying the account
if(Schema.SobjectType.Account.isaccessible()){
System.debug('User has permission to query Account Records');
}
You might see different logs based on object permission of logged in user profiles. Below is the output which I got after executing the above code.
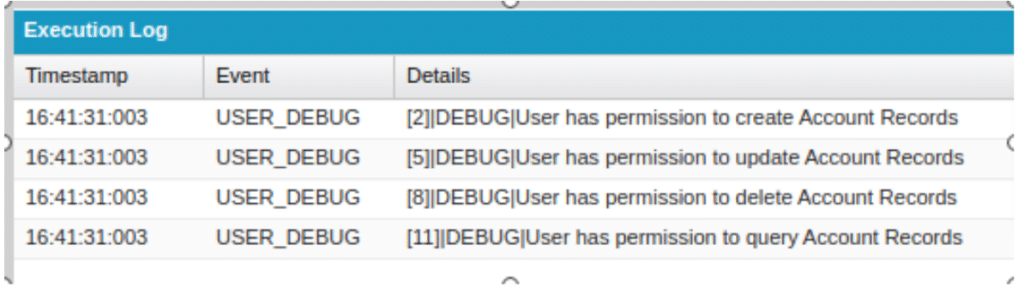
By looking at the above code it is clear that I have permission to create, edit, delete and query account objects.
In above code Schema.SobjectType.Account refers to metadata information of account object. Schema is a namespace in Salesforce Apex which provides classes and methods for accessing metadata information of objects, fields in salesforce schema. SobjectType is a class inside Schema namespace used to represent different types of salesforce objects. Account is the specific sobject being referenced here.
iscreateble() is a method provided by SobjectType class it returns a boolean value indicating whether the current user has permission to create new records of the specified object in our example Account object. If the user has permission, the method returns true otherwise false.
isupdateable(), isdeletable() and isaccessible() is used to check update, delete and query permission respectively.
Learn more about dynamic Apex.
I hope now you know why we need to check object permission along with how to do that using apex. Since you are here can you tell me what are the changes we need to do in above code to check delete permission of address custom object.
Post the answer in the comment box below.