In this short article, you will be introduced to Exception Handling in Apex.
What is an Exception?
Let’s start understanding Exception in Apex by executing below code snippets. You can execute the code from the Anonymous window.
Integer division_result = 10/0;
system.debug(division_result);
You will get an error like the one in the image below.
It says that System.MathException: Divide by 0 which is correct because in our example we are trying to divide 10 by 0 which is impossible and hence we got an error which halted execution of our code therefore we don’t see a debug log as well.
In any programming language, whenever an error occurs or we get an exception, the execution of code will stop, and we will see an error message. So, an exception can be defined as an event that disrupts the normal flow of program execution.
Learn about Different types of Exceptions in Salesforce.
Let’s learn how to handle these exceptions and continue executing our code.
Handle Exceptions using try-catch
Execute the code snippet below from the anonymous window.
try{
Integer division_result = 10/0;
system.debug(division_result);
}catch(Exception ex){
System.debug('An exception occured');
}
This time, you will not see the error message popup that we saw while executing the earlier example. Now open the latest log and click on debug only.
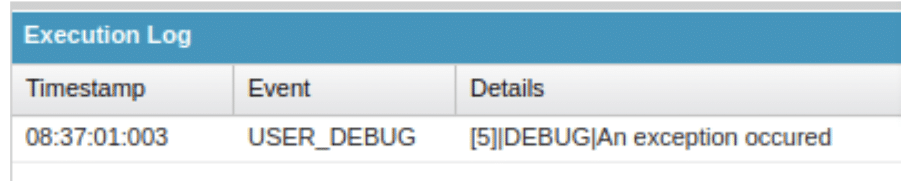
So what we did here is move the code that gave an exception inside the try block and add a catch block, which will execute when an exception occurs in the try block.
In short we can use try block to write the code which can give errors and inside catch block we handle those exceptions to continue normal flow of code execution. Let’s execute one more code snippet to handle specific exceptions
try{
Integer division_result = 10/0;
system.debug(division_result);
}
catch(MathException ex){
System.debug('Math Exception occured '+ex.getMessage());
}
catch(Exception ex){
System.debug('Exception occured');
}
Now open the latest log and click on debug. Only you will see the log like the image below.
Lets understand what are the changes we did in the code. First we added one more catch block. Yes that is right you can add multiple catch blocks for a single try block. Here we have added a more specific exception which is MathException and also we have updated the debug log to print the error message as well using ex.getMessage() method which is why you saw Divide by 0 in log.
Also code inside the next catch block was not executed because whenever there are multiple catch blocks then the block with more specific Exception is executed and if our code doesn’t contain a specific block then the block with Exception will always execute.
We already learned about the getMessage() method. Let’s learn about the remaining methods as well. Execute below code from Anonymous window.
try{
Integer division_result = 10/0;
system.debug(division_result);
}
catch(Exception ex){
System.debug('Exception type caught: ' + ex.getTypeName());
System.debug('Message: ' + ex.getMessage());
System.debug('Cause: ' + ex.getCause());
System.debug('Line number: ' + ex.getLineNumber());
System.debug('Stack trace: ' + ex.getStackTraceString());
}
This is the output that you will see when you open the log.
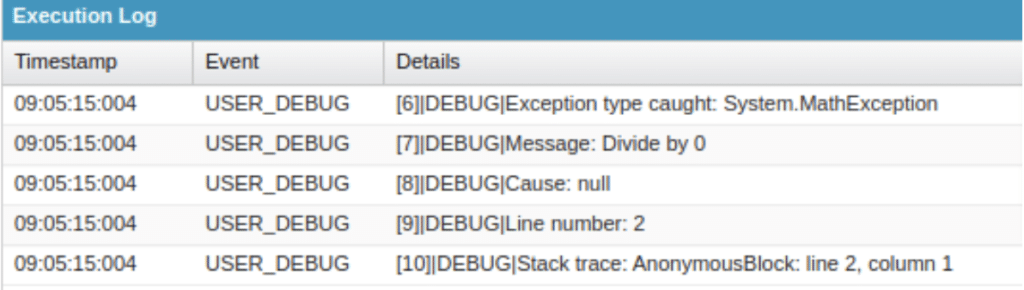
You can learn more about these methods in detail from the Apex Developer Guide.
Finally
Let’s execute one more piece of code.
try{
Integer division_result = 10/0;
system.debug(division_result);
}
catch(Exception ex){
System.debug('Exception type caught: ' + ex.getTypeName());
System.debug('Message: ' + ex.getMessage());
System.debug('Cause: ' + ex.getCause());
System.debug('Line number: ' + ex.getLineNumber());
System.debug('Stack trace: ' + ex.getStackTraceString());
}
finally{
System.debug('This will always execute.');
}
Here, we have added a final block, which will always execute irrespective of whether an error occurs or not. Here is the output which you will see in debug logs.
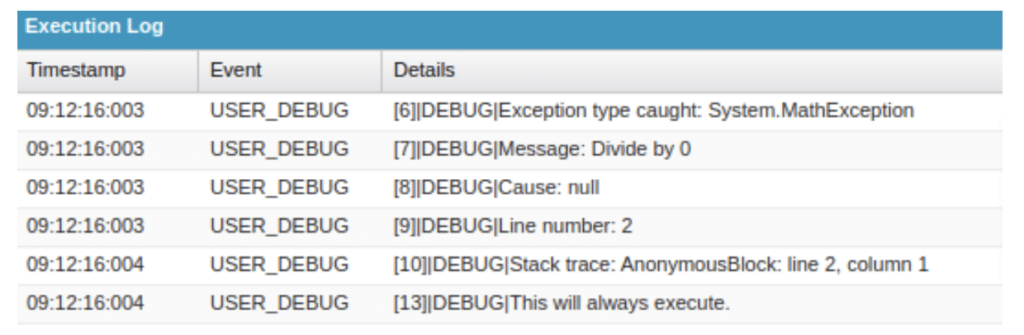
We already learned about MathException but there are other built-in exceptions such as DMLException, ListException, QueryException, SObjectException, etc, for a complete list of exception types check this Apex Reference Guide.
Since you are here, I have a question for you, which you might see in your next Salesforce interview as well.
Which type of exception cannot be caught by a try-catch block? Leave a comment if you know the answer.
I know this was just an example and wasn’t a direct recommendation, but I think it’s important to specify that using try catch with only a debug statement in the catch (aka swallowing the exception) is dangerous. a catch or finally block should almost always handle the error, not just log it.