Join us and learn about the Builder pattern in Salesforce. In this session will talk about how and when to implement Builder pattern in Apex.
What is the Builder pattern?
The builder pattern is a creational pattern – it helps you to instantiate objects in a particular way. This pattern allows you to construct complicated objects step by step. Builders help to populate immutable private variables at construction without multiple position-based constructor arguments.
Builder Pattern Class Diagram
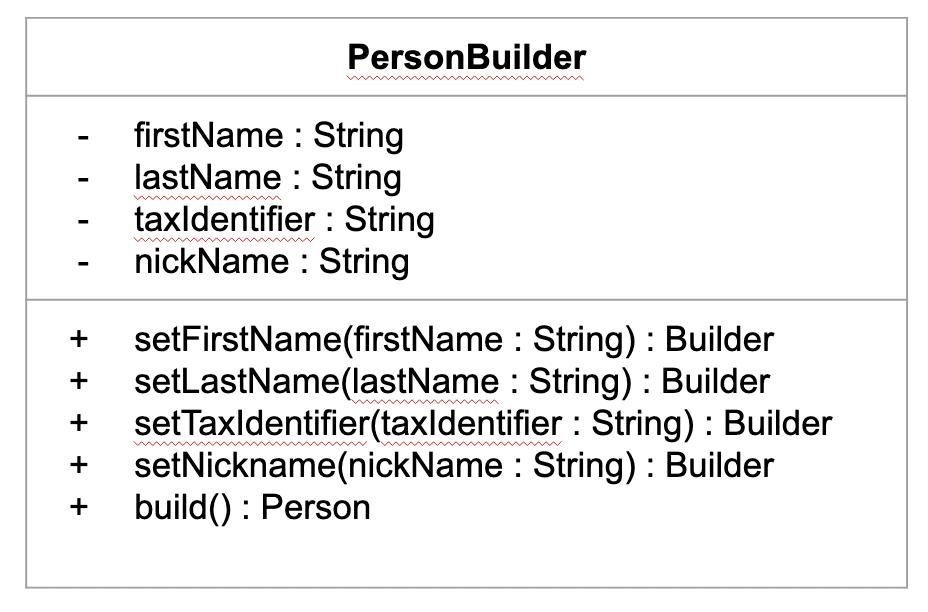
When should we use the Builder pattern?
Use the builder pattern when you have a constructor which sets more than two properties in the object to be built.
The builder pattern doesn’t enable more dynamic application behavior; its purpose is to increase readability when instantiating objects.
Problematic Code
In below code we have to many parameter with same data type
public class Person {
private final String firstName;
private final String lastName;
private final String taxIdentifier;
private final String nickname;
public Person(
String firstName,
String lastName,
String taxIdentifier,
String nickname
) {
this.firstName = firstName;
this.lastName = lastName;
this.taxIdentifier = taxIdentifier;
this.nickname = nickname;
}
}
How to instance the above class.
Person Obj = new Person(
'Apex',
'Hours',
'123-456-7890',
'apexHours'
);
Multiple positional arguments can make it a little challenging to understand for the future readers. more properties -> less readable
Fluent Interface
public class PersonBuilder {
private String firstName, lastName, taxIdentifier, nickname;
// don’t format your code like this
public builder setFirstName(String firstName) {
this.firstName = firstName;
return this;
}
public builder setLastName(String lastName) {
this.lastName = lastName;
return this;
}
// ...
public Person build() {
return new Person(
this.firstName, this.lastName, this.taxIdentifier, this.nickname
);
}
}
Before and After
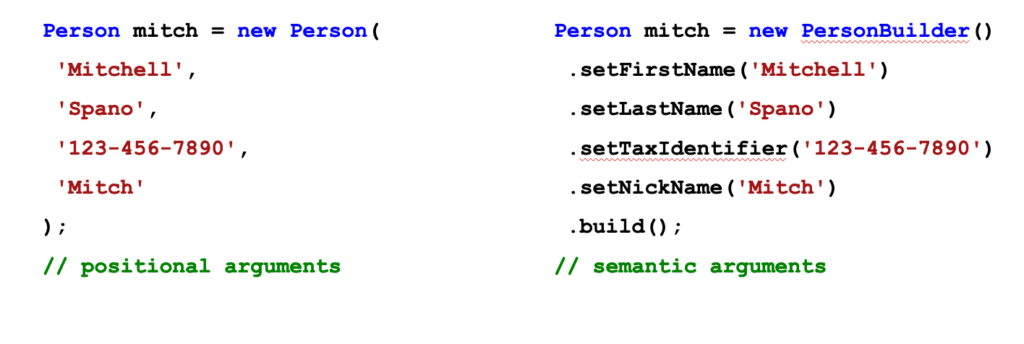
How to implement Builder pattern in Apex.
Example of Builder pattern in Salesforce
Helpful with JSON Construction
public Database.Error createError(
StatusCode statusCode,
String message,
List<String> fields
) {
Map<String, Object> result = new Map<String, Object>{
'message' => message,
'statusCode' => statusCode,
'fields' => fields
};
return (Database.Error) JSON.deserialize(
JSON.serialize(result),
Database.Error.class
);
}
Before and After
// before
Database.Error error = createError(
StatusCode.CUSTOM_APEX_ERROR,
'Oh no mr. Bill!',
new List<String>{ 'Name', 'Id' }
);
// after
Database.Error error = new ErrorBuilder()
.setStatusCode(StatusCode.CUSTOM_APEX_ERROR)
.setMessage('Oh no mr. Bill!')
.setFields(new List<String>{ 'Name', 'Id' })
.build();
Summary
I hope this session will help you understand the builder pattern. Check our other Apex Design pattern too.
What is the ‘builder’ class in this line “public builder setFirstName(String firstName)”? Should it be changed to PersonBuilder?